In this article we will use two ESP8266 board to show internal and external temperature in an OLED screen using Espruino and some JavaScript code.
The internal board will:
- read the temperature and humidity from a DHT22 sensor
- receive the external temperature and pressure from the external ESP board
- show all the information in the OLED screen
The external board will:
- read the temperature and barometric pressure from a BMP085 sensor
- send the temperature and the pressure to the internal board using a WiFi connection
In this article we will reuse the wiring and the code we wrote in the previous articles about the ESP8266 board.
Wiring
The internal board is wired to the DHT22 sensor and the OLED screen:
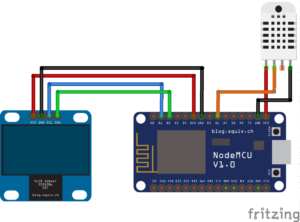
The external board will be connected to the BMP085 sensor:
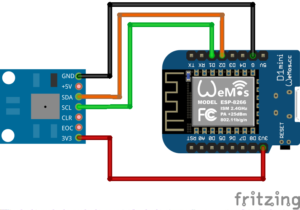
Internal board code
The internal board gets the internal temperature using the DHT22 sensor and print it in the OLED screen. The board prints also the external board information, we keep such information in an externalData variable.
var dht = require("DHT22").connect(NodeMCU.D4);
var g;
var externalData;
function drawData(temperature, humidity) {
g.clear();
g.setColor(5);
g.drawString("INT", 0, 2);
g.drawString("T: " + temperature + " C", 0, 20);
g.drawString("H: " + humidity + " %", 0, 40);
g.drawString("EXT", 62, 2);
if (externalData) {
g.drawString("T: " + externalData.temperature.toFixed(2) + " C", 62, 20);
var pressureInBar = externalData.pressure / 100000;
g.drawString("P: " + pressureInBar.toFixed(2) + " bar", 62, 40);
} else {
g.drawString("N/A", 62, 20);
}
g.flip();
}
function readTemperature() {
dht.read(function (info) {
drawData(info.temp, info.rh);
});
}
function setupGraphics() {
require("Font8x12").add(Graphics);
g.setFont8x12();
g.drawString("Ready", 2, 2);
g.flip();
setInterval(readTemperature, 2000);
}
function setupOLED() {
I2C1.setup({scl:NodeMCU.D1, sda:NodeMCU.D2});
g = require("SSD1306").connect(I2C1, setupGraphics);
}
But how the externalData variable get updated? Through a socket server that listen for new connection on port 1234. When a connection is established the server read the sent data.
var wifi = require("Wifi");
function handleClient(socket) {
socket.on('data', function(data) {
console.log('external data: ', data);
externalData = JSON.parse(data);
socket.end();
});
}
function setupServer() {
var net = require("net");
var server = net.createServer(handleClient);
server.listen(1234);
}
function connectWiFi() {
wifi.connect("***WIFI*SSID***", {password:"***WIFI*PASSWORD***"},
setupServer
);
}
function onInit() {
setupOLED();
connectWiFi();
}
save();
The complete code is available as attachment. Time to setup the external board.
External board code
The external board reads the temperature and the barometric pressure from the BMP085 sensor and send it to the internal board using a TCP connection.
var bmp;
function setupBmpSensor() {
I2C1.setup({scl:NodeMCU.D1, sda:NodeMCU.D2});
bmp = require("BMP085").connect(I2C1);
}
var wifi = require("Wifi");
function sendDataToServer(data) {
var net = require("net");
net.connect({host: "192.168.1.105", port: 1234}, function(socket){
var json = JSON.stringify(data);
socket.end(json);
});
}
function getAndSendData() {
bmp.getPressure(sendDataToServer);
}
function setupDataPolling() {
setInterval(getAndSendData, 2000);
}
function connectWiFi() {
wifi.connect(
"***WIFI*SSID***",
{password:"***WIFI*PASSWORD***"},
setupDataPolling
);
}
function onInit() {
setupBmpSensor();
connectWiFi();
}
save();
The result
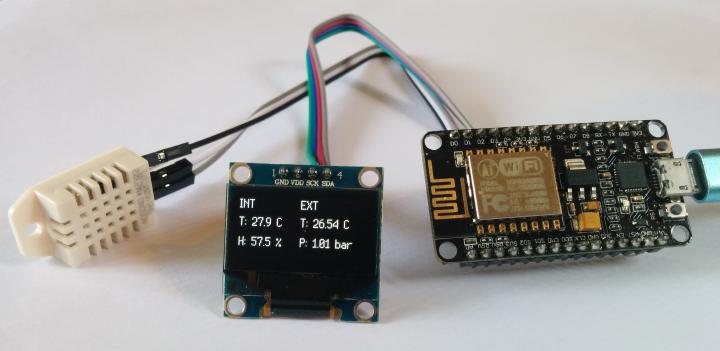
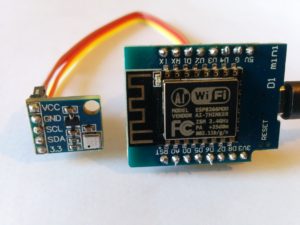
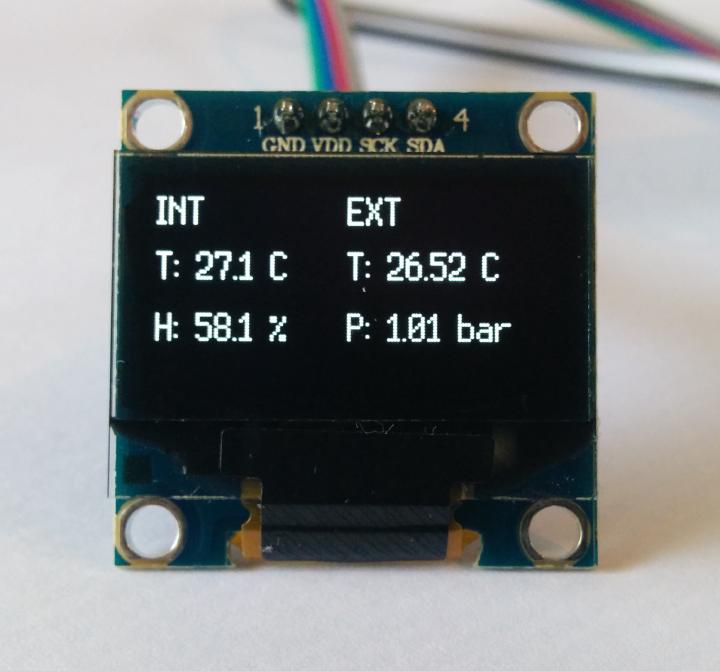