In this short tutorial we will read the barometric pressure from the BMP085 sensor using an ESP8266 board and Espruino with some Javascript code.
Wiring
Our BMP085 sensor communicates through an I2C connection, so we need to connect the SCL pin to the corresponding D1 pin and the SDA pin to the corresponding D2 pin in the ESP board. The other two pins are dedicated to the power.
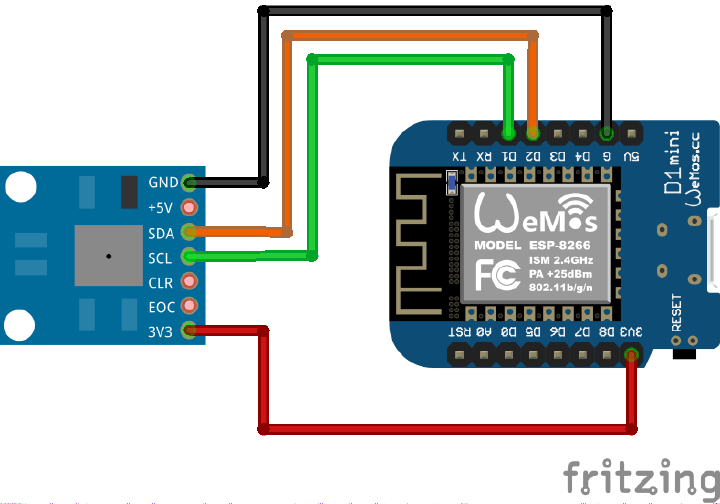
Code
To read both pressure and temperature we will use the BMP085 module of Espruino. After the I2C connection is setup we initialize the BMP085 module, then we start polling and printing the sensor information.
I2C1.setup({scl:NodeMCU.D1, sda:NodeMCU.D2});
var bmp = require("BMP085").connect(I2C1);
function readPressure() {
bmp.getPressure(function(info) {
console.log("Pressure: " + info.pressure + " Pa");
console.log("Temperature: " + info.temperature + " C");
});
}
setInterval(readPressure, 2000);
Here the result:
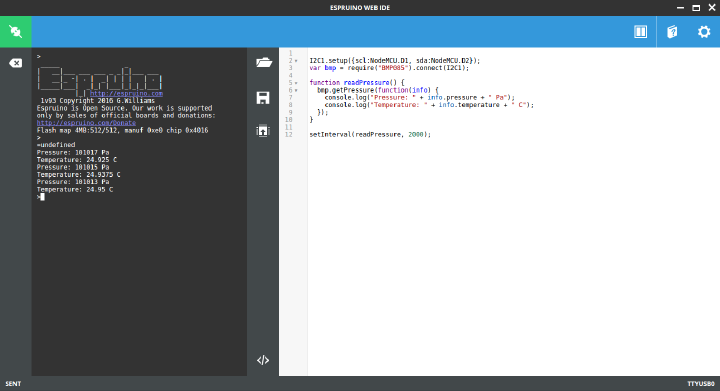