In this short article I will show you how to read the temperature and the relative humidity from a DHT22 sensor and display it in a 128x64 OLED display using an ESP8266 board with Espruino and JavaScript running on it.
Wiring
The DHT22 has 4 pins, only three used: two for the power and one for the data. We will connect the power pins to the corresponding pins on the ESP8266 board and the data pin to one of the GPIO pin, D7 in our schema.
The display is a SSD1306 with a resolution of 128x64 pixels and I2C communication. The display has four pins, two for the power and two for the I2C. We connect the I2C pin to the corresponding D1 e D2 pins in the ESP8266 board.
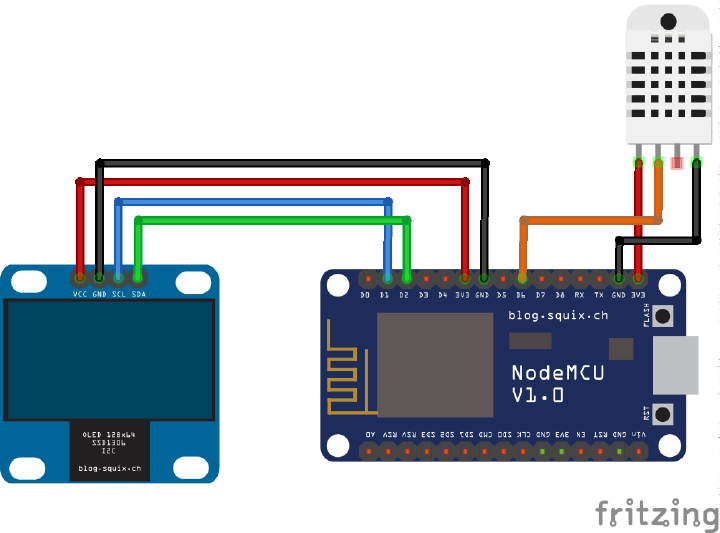
Code
Espruino provides a module for reading the temperature and the relative humidity from a DHT22 sensor. We will use it to read both the values from the connected sensor.
Then we will use the I2C module combined to the SSD1306 module to show the collected information in the display.
We will poll the sensor every 2 seconds and update the display consequently.
var dht = require("DHT22").connect(NodeMCU.D7);
function drawData(temperature, humidity) {
g.clear();
g.drawString("T: " + temperature + " C", 2, 2);
g.drawString("H: " + humidity, 2, 20);
g.flip();
}
function readTemperature() {
dht.read(function (info) {
drawData(info.temp, info.rh);
});
}
function setupGraphics() {
require("Font8x16").add(Graphics);
g.setFont8x16();
g.drawString("Ready", 2, 2);
g.flip();
setInterval(readTemperature, 2000);
}
I2C1.setup({scl:NodeMCU.D1, sda:NodeMCU.D2});
var g = require("SSD1306").connect(I2C1, setupGraphics);
save();
The final result:
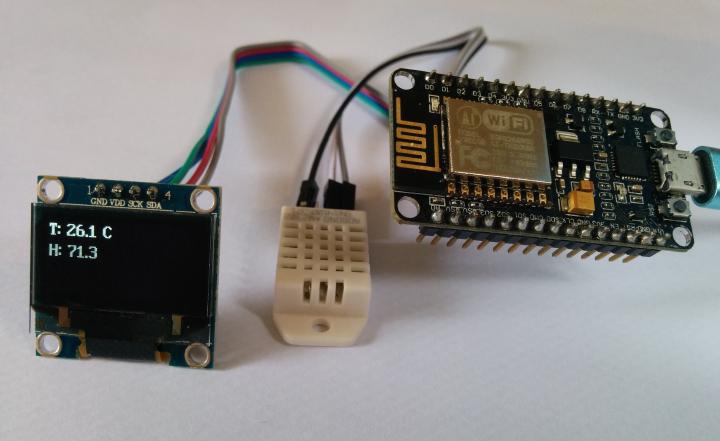