This post will show you how a Dart program running in a Raspberry Pi board can communicate to an Arduino board.
To show it we will send a text message from a Dart program running in a Raspberry Pi and we will visualize it in an LCD controlled by an Arduino board.
HARDWARE SETUP
The main components are:
- A Raspberry Pi Model B revision 1.0 running Raspbian 2014-09-09
- An Arduino Duemilanove
- A 16x4 LCD
- breadboard, wires and cables
The Raspberry Pi board is connected to the Arduino board through an USB cable.
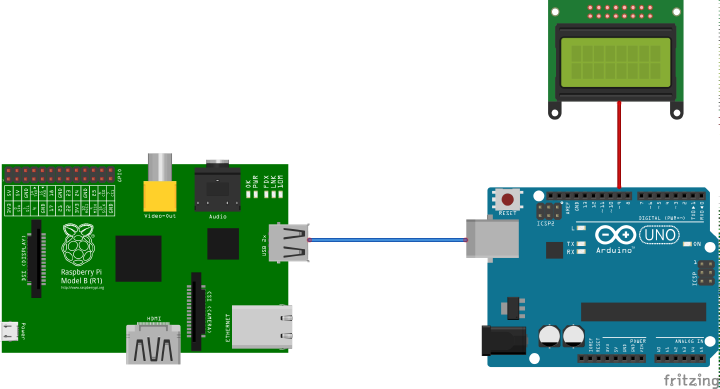
The LCD is wired to the Arduino board following the schema proposed in the Liquid Crystal Library example.
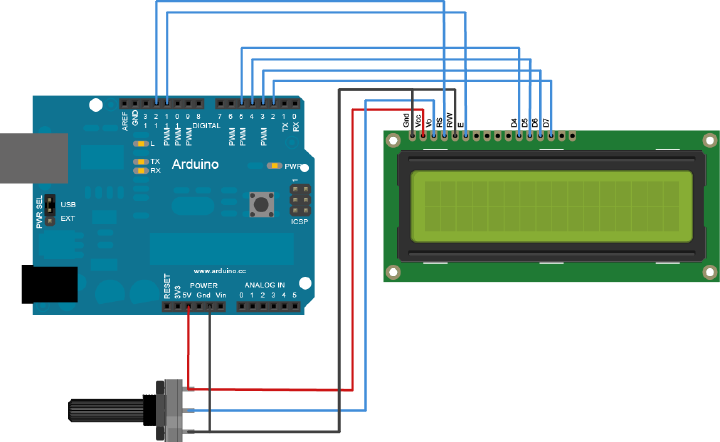
SOFTWARE
For the Arduino side I wrote a simple sketch using the Liquid Crystal library.
In the loop block the sketch reads a String from the USB and writes it to the LCD. The sketch replies to the Raspberry Pi board with an “Hello Pi!” message.
#include <LiquidCrystal.h>
LiquidCrystal lcd(12, 11, 5, 4, 3, 2);
void setup() {
//communication setup
Serial.begin(9600);
//LCD setup
lcd.begin(16, 4);
lcd.clear();
lcd.print("Listening...");
}
void loop() {
String read = Serial.readString();
if (read.length()!=0) {
lcd.clear();
lcd.print(read);
Serial.print("Hello Pi!");
}
}
For the Raspberry Pi side I wrote a Dart program that sends a message to the USB port using the serial_portal package.
The program initializes the USB port and sets a listener for the incoming bytes in order to write them in the console. After the setup it opens the USB port and sends the “Hello Dart!” message.
import 'package:serial_port/serial_port.dart';
import 'dart:async';
import 'dart:io';
main(){
var arduino = new SerialPort("/dev/ttyUSB0");
arduino.onRead.listen((List<int> bytes){bytes.forEach(stdout.writeCharCode);});
arduino.open().then((_) {
new Timer(new Duration(seconds:3), (){
arduino.writeString("Hello Dart!");
print('sent "Hello Dart!"');
});
});
}
To run the program in the Raspbian environment I had to compile the Dart SDK.
In the screenshot you can see the Dart program running in the Raspbian terminal.
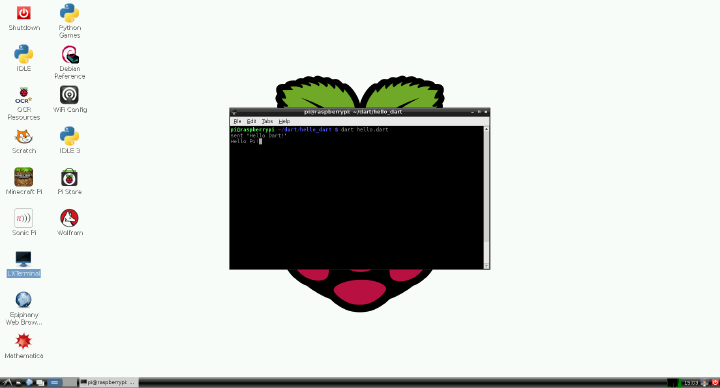
Here the source code: Dart-RaspberryPi-Arduino.zip